how to pass data between components in react js
9 March 2024
techtipsall.com
Welcome to my blog techtipsall. in this blog we will discuss about in detail how to pass data between components in react js.
Summary: When we create a React application, we need components to create the application. We also have to interlink the components with each other. Because data has to be exchanged in any application.’
When we talk about exchanging data from one component to another, React provides us some functionality so that we can easily pass data from one component to another.
Below we will explain how data is passed.
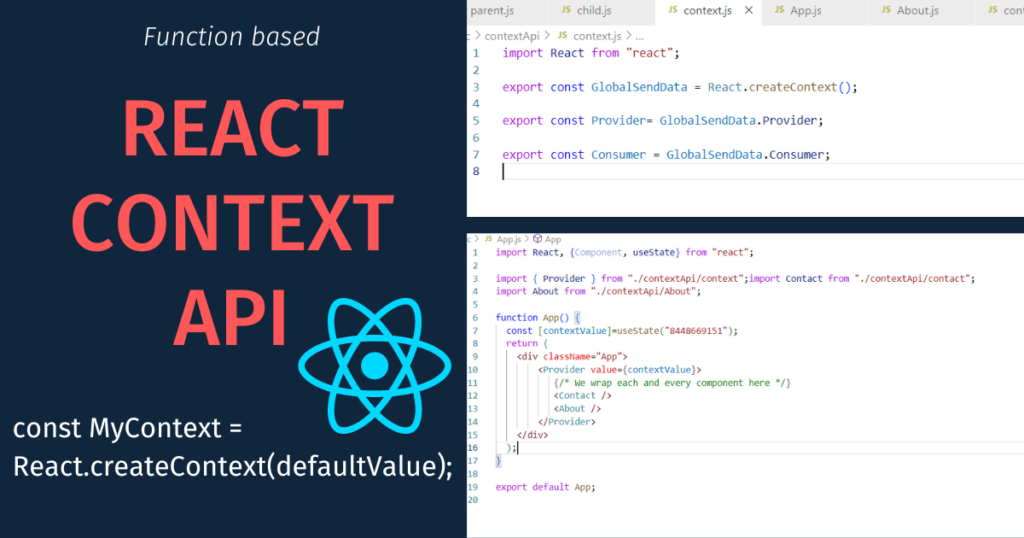
There are multiple ways for data passing from one component to another component.
- Passing Data from Parent component to Child component.
- Passing Data from Child component to Parent Component.
- Passing Data using Context API.
- Passing Data using Redux.
- Combination of the above two methods (callback and use of props).
Steps to create the application
Step 1: open the terminal and choose the project location
Step 2: And then type command
npx create-react-app techtipsallproject
Step 3: then change directory
cd techtipsallproject
npm start
Project Structure
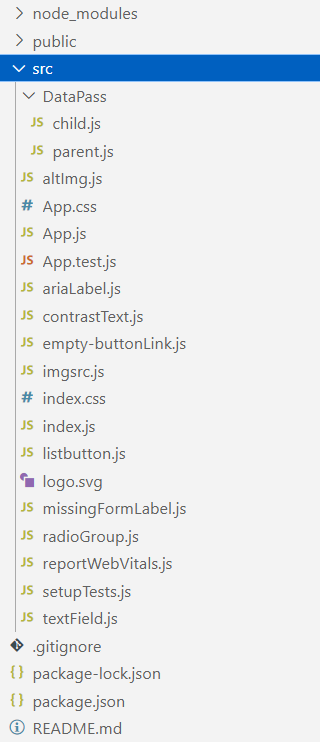
How to pass Data from Parent component to Child component in react js.
We use props to send data from parent component to child component. This props data is sent by the parent component and cannot be changed by the child component.
Here’s an example of how to pass data from parent component to child component.
Parent.js
import React from 'react'; import Child from './child'; const persons = [ { "firtName": 'john', "emp_id": 'Sus1567', "Organization": 'Smart energy water', "post": "Module Lead" }, { "firtName": 'sohan', "emp_id": 'Sus1567', "Organization": 'Smart energy water', "post": "Module Lead" }, ] const Parent = () => { return ( <div className='parent-data'> Data pass from parent to child component by using props <Child fromParentData="We are comming data from parent" personInfo={persons} /> </div> ) } export default Parent;
Child.js
import React from 'react'; const Child = (props) => { console.log(props); let ObjefctData = props.personInfo.map((info, index) => { return ( <tr key={index}> <td>{info.firtName}</td> <td>{info.emp_id}</td> <td>{info.Organization}</td> <td>{info.post}</td> </tr> ) }) return ( <div className='child-data border-top'> <h1> child component area</h1> <h6> {props.fromParentData} </h6> <table border="1" cellPadding={20}> <thead> <th>firtName</th> <th>emp_id</th> <th>Organization</th> <th>post</th> </thead> <tbody> {ObjefctData} </tbody> </table> </div> ) } export default Child;
Conclusion:
As you saw in top example child component imported in parent component. and as the props “fromParentData” and “personInfo” both are props sent data to child component.
<Child fromParentData=”We are comming data from parent” personInfo={persons}/>
We can receive the sent props in the child component like this.
const Child = (props) => { console.log(props)return(<><p>{props.fromParentData}</p><p>{props.personInfo}</p></>)}
We can make this code even simpler by destructuring the “fromParentData” and “personInfo” props like so
const Child = ({fromParentData,personInfo}) => { console.log(props)return(<><p>{fromParentData}</p><p>{personInfo}</p></>)}
Result:
Passing Data from Child component to Parent Component in react Js.
To pass data from child component to parent component, we have to create a callback function and then pass the callback function to the child component and pass props to it.
And after this callback function has to be called in the parent component. In this way the parent component gets the data from the child component.
We will understand through examples.
Approach:
- Create a callback function in the Parent component.
- As a props pass the callback function to the child from the parent component.
- Using props in child components calls the parent callback function and passes data to the parent component as arguments.
Parent.js
import React, { useState } from 'react'; import Child from './child'; const Parent =()=>{ const [holdChildData,setHoldChildData]=useState("right now parent data") const fetchChildDataFun=(data)=>{ setHoldChildData(data) } return( <div className='parent-data'> <h1> Parent Component</h1> Data coming from child component to parent component <h3> {holdChildData}</h3> <Child childToParent={fetchChildDataFun}/> </div> ) } export default Parent;
CallBack Function: A callback function is a function passed in to another function as an argument, which is then invoked inside the outer function to complete an action
child.js
import React from 'react'; cons Child = (props) => { console.log(props); const dataSendFun = () => { props.childToParent("data is coming from child") } return ( <div className='child-data border-top'> <h1> Child component data</h1> <button type="button" onClick={dataSendFun}> Data pass to parent onclick </button> </div> ) } export default Child;
Result Here:
Passing Data using Context Api in react js
React context api is main used for solve the problem of prop drilling (also called “Threading”).
With the help of Context API, we can pass any type of data directly to any component. We do not require drilling props.
How to Get Started with the Context API
To start using the Context API in your applications, We have to pay attention to some points given below.
- React.createContext
- Context.provider
- Context.consumer
1. Create a Context Object
First of all, you create a context object using the createContext.
it’s a predefined function of react library. createContext object hold the data that we want to share this data to across in component related to our Application.
Ex:
import React from "react"; export const GlobalSendData = React.createContext();
2. Wrap Components with a Provider
Once you have created the context object, then you have to wrap all
the components with provider to the components to which you want to send data.
Provider components keep value as the props. You can send any type of data in such props value.
or
Provider provides the value to the child component.
<Provider value={"context value"}> {/* We wrap each and every component here */} <Contact /> <About /> </Provider>
In top example You can see that all the components which need to send data have been wrapped by the provider. or You can also simply wrap the parent component of all these components so that you can easily receive any type of data sent by the provider.
3. Consume the Context
After creating the Context Object and Provider, we have to need the consume the Context in other components. for consume the context from provider we will have to used “UseContext” hook from React.
Provider: provider is provides the value of the component.
Consumer: consumer is consuming the value of the component
Here’s an example of how to pass data using context api.
Step 1: We will create contextApi folder
Step2: And then we will create context.js, contact.js and about.js file
As you see in screenshot
We will create a context.js file in src folder.
import React, { createContext } from "react"; export const GlobalSendData = createContext(); export const Provider= GlobalSendData.Provider; export const Consumer = GlobalSendData.Consumer;
in the top code example we are importing createContext from predefined React and using it to create new context object named “GlobalSendData”. after that exporting the context object so that we can use it on other components of our application.
Ex:- export const GlobalSendData = React.createContext();
And then after we have created a provider for sending data and also exported.
Ex:- export const Provider= GlobalSendData.Provider;
and then created a consumer object and also exported to it.
Ex: export const Consumer = GlobalSendData.Consumer;
We will create app.js file in src folder for sending data.
import React, { Component, useState } from "react"; import { Provider } from "./contextApi/context"; import Contact from "./contextApi/contact"; import About from "./contextApi/About"; function App() { const [contextValue] = useState("8448669151"); return ( <div className="App"> <Provider value={contextValue}> {/* We wrap each and every component here */} <Contact /> <About /> </Provider> </div> ); } export default App;
And the we have created contact.js file in src folder for consume data from provider
import React, {useContext} from "react"; import {GlobalSendData} from "./context"; const Contact = () => { const Valuedata = useContext(GlobalSendData); return ( <> <h1>Conatct Us page</h1> <h3> We are fetching global data using context api As you see mobile number i will be fetch from globally {Valuedata} </h3> </> ) } export default Contact;
And same we will create About.js in src folder for consume data sented by provider.
import React, {useContext } from "react"; import { GlobalSendData } from "./context"; const About =()=>{ const mobileNumber =useContext(GlobalSendData); return( <> <h1> My mobile number is {mobileNumber}</h1> </> ) } export default About;